Rock, Paper and Scissor Game.
All About working of rock, paper and scissor game..
How this game works?
- First we have to detect hand using cvzone HandDetector module.
- Now we have to initialize the back ground image and read the realtime image using webcam.
-
Here to fit our webcam window to the right side box of back ground image we have to do some calculation.
-
Main concept of the game is to detect the list of figers index which is either raised or not and given by 0 if finger is not raised and 1 if finger is raised.
- [0,0,0,0,0] = Rock(All the finger are not raised)
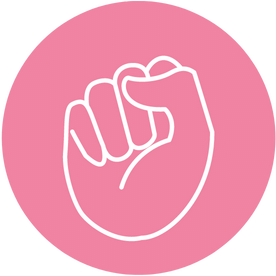
-
[1,1,1,1,1] = Paper(All finger is raised)
-
[0, 1, 1, 0, 0] = Scissor(second and third finger is raised others are kept as 0)
- Now we will create a random number for AI wins and select a ramdom images according to number.
- For Players to win there should come rock at player side and scissor at AI side or scissor at player side and paper at AI side or paper at player side and rock at AI side. Vice versa for AI to win.
- We do calculations to fit AI images score to the backgroud images and we do simple pixel calculation to perfectly fit the score in images.
- We also set condition as when we press the s key game will start with the timer of 3 sec.
import random
import cv2
import cvzone
from cvzone.HandTrackingModule import HandDetector
import time
cap = cv2.VideoCapture(0)
cap.set(3, 640)
cap.set(4, 480)
detector = HandDetector(maxHands=1)
timer = 0
stateResult = False
startGame = False
scores = [0, 0] # [AI, Player]
while True:
imgBG = cv2.imread("Resources/BG.png")
success, img = cap.read()
imgScaled = cv2.resize(img, (0, 0), None, 0.875, 0.875)
imgScaled = imgScaled[:, 80:480]
# Find Hands
hands, img = detector.findHands(imgScaled) # with draw
if startGame:
if stateResult is False:
timer = time.time() - initialTime
cv2.putText(imgBG, str(int(timer)), (605, 435), cv2.FONT_HERSHEY_PLAIN, 6, (255, 0, 255), 4)
if timer > 3:
stateResult = True
timer = 0
if hands:
playerMove = None
hand = hands[0]
fingers = detector.fingersUp(hand)
if fingers == [0, 0, 0, 0, 0]:
playerMove = 1
if fingers == [1, 1, 1, 1, 1]:
playerMove = 2
if fingers == [0, 1, 1, 0, 0]:
playerMove = 3
randomNumber = random.randint(1, 3)
imgAI = cv2.imread(f'Resources/{randomNumber}.png', cv2.IMREAD_UNCHANGED)
imgBG = cvzone.overlayPNG(imgBG, imgAI, (149, 310))
# Player Wins
if (playerMove == 1 and randomNumber == 3) or \
(playerMove == 2 and randomNumber == 1) or \
(playerMove == 3 and randomNumber == 2):
scores[1] += 1
# AI Wins
if (playerMove == 3 and randomNumber == 1) or \
(playerMove == 1 and randomNumber == 2) or \
(playerMove == 2 and randomNumber == 3):
scores[0] += 1
imgBG[234:654, 795:1195] = imgScaled
if stateResult:
imgBG = cvzone.overlayPNG(imgBG, imgAI, (149, 310))
cv2.putText(imgBG, str(scores[0]), (410, 215), cv2.FONT_HERSHEY_PLAIN, 4, (255, 255, 255), 6)
cv2.putText(imgBG, str(scores[1]), (1112, 215), cv2.FONT_HERSHEY_PLAIN, 4, (255, 255, 255), 6)
# cv2.imshow("Image", img)
cv2.imshow("BG", imgBG)
# cv2.imshow("Scaled", imgScaled)
key = cv2.waitKey(1)
if key == ord('s'):
startGame = True
initialTime = time.time()
stateResult = False